C Program to Find Factorial Using Recursive Function
A recursive function is a function defined in terms of itself via self-calling expressions. This means that the function will continue to call itself and repeat its behavior until some condition is satisfied to return a value.
Factorial Using Recursive Function
/*
Program: Factorial of a given number using recursive function.
Author: Codesansar
Date: Jan 22, 2018
*/
#include<stdio.h>
#include<conio.h>
int fact(int n); /* Function Definition */
void main()
{
int num, res;
clrscr();
printf("Enter positive integer: ");
scanf("%d",&num);
res = fact(num); /* Normal Function Call */
printf("%d! = %d" ,num ,res);
getch();
}
int fact(int n) /* Function Definition */
{
int f=1;
if(n <= 0)
{
return(1);
}
else
{
f = n * fact(n-1); /* Recursive Function Call as fact() calls itself */
return(f);
}
}
Output
Enter positive integer: 5↲ 5! = 120
Working of above recursive example can be illustrated using following diagrams:
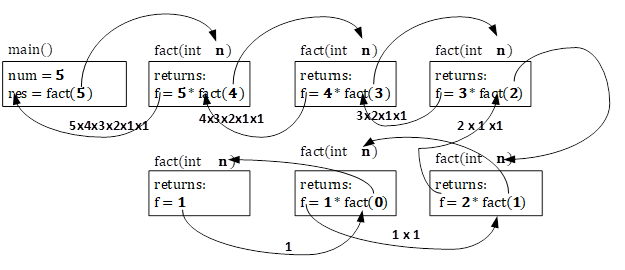
Explanation:
/* ... */
: A multi-line comment providing program details including purpose, author, and date.#include<stdio.h>
and#include<conio.h>
: Include necessary header files for input-output and console manipulation.int fact(int n);
: Declare the prototype of thefact
function.void main()
: Start of themain
function.int num, res;
: Declare variables to store user input and the factorial result.clrscr();
: Clear the console screen.printf("Enter positive integer: ");
: Prompt the user to enter a positive integer.scanf("%d", &num);
: Read user input and store it innum
.res = fact(num);
: Call thefact
function and assign the result tores
.printf("%d! = %d", num, res);
: Display the input number and its factorial.getch();
: Wait for user input to continue.int fact(int n)
: Start of thefact
function.int f = 1;
: Initialize a local variablef
to store the factorial result.if (n <= 0)
: Check ifn
is less than or equal to 0.return 1;
: Return 1 ifn
is negative or 0.else
: Execute the following block ifn
is positive.f = n * fact(n - 1);
: Calculate factorial recursively usingf = n * fact(n - 1)
.return f;
: Return the calculated factorial value.
Note: The use of conio.h
and its functions may not be compatible with all compilers and systems.