Python Program to Plot Coordinates on a Plane
This article explains about plotting coordinates on a plane in Python programming language.
To plot coordinates in Python, we use pyplot
module from matplotlib
library. Pyplot module has plot()
methods to plot coordinates.
Plotting Single Point
Following python program plots single point on a plane.
# Python program to plot coordinates on a plane
# Plotting single point
from matplotlib import pyplot as plt
# Coordinates
x = 2
y = 4
# Plotting
plt.plot(x,y,'bo')
# Displaying grid
plt.grid()
# Controlling axis
plt.axis([-8, 8, -8, 8])
# Adding title
plt.title('Single Point Plot')
# Displaying plot
plt.show()
Output
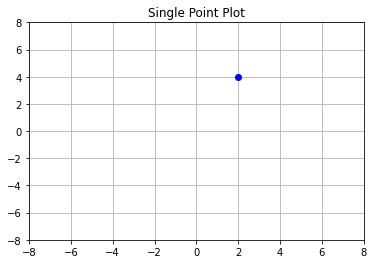
In above program, third argument i.e. bo
in plt.plot(x,y, 'bo')
represents - blue color circle are used to mark the Coordinate.
Plotting Multiple Points
Following python program plots multiple point on a plane.
# Python program to plot coordinates on a plane
# Plotting multiple point
from matplotlib import pyplot as plt
# Coordinates
x = [2, -4, 6, -8]
y = [4, 3, -8, 10]
# Plotting
plt.plot(x,y,'r*')
# Displaying grid
plt.grid()
# Controlling axis
plt.axis([-12, 12, -12, 12])
# Adding title
plt.title('Multiple Point Plot')
# Displaying plot
plt.show()
Output
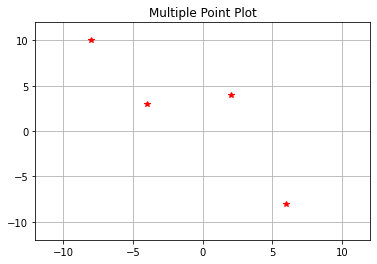
In above program, third argument i.e. r*
in plt.plot(x,y, 'r*')
represents - red color star are used to mark the Coordinate.