Python Program to Plot Logarithmic Curve Using MatplotLib & Numpy
This Python program plots logarithmic curve using numpy and matplotlib library.
Python Source Code: Logarithmic Curve
# Importing Required Libraries
import numpy as np
import matplotlib.pyplot as plt
# Generating x-axis data using arange function from numpy
xdata = np.arange(0.0001,100, 0.01)
constant = 0.8
# Finding y-axis for each x-data using log(xdata)
ydata = np.log(xdata)
# Plotting xdata vs ydata using plot function from pyplot
plt.plot(xdata, ydata)
# Settng title for the plot in blue color
plt.title('Logarithmic Curve', color='b')
# Setting x axis label for the plot
plt.xlabel('X'+ r'$\rightarrow$')
# Setting y axis label for the plot
plt.ylabel('Y '+ r'$\rightarrow$')
# Showing grid
plt.grid()
# Highlighting axis at x=0 and y=0
plt.axhline(y=0, color='k')
plt.axvline(x=0, color='k')
# Finally displaying the plot
plt.show()
Output
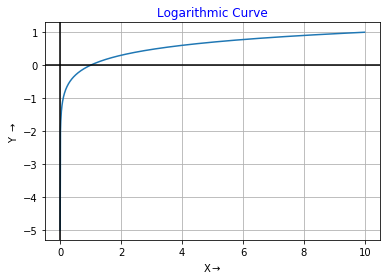