Beautiful Grid Layout in Flutter Using GridView Widget
Grid layout is very useful to display different elements of an application. With flutter designing grid layout is as simple as ABC using GridView
widget. In this tutorial we explain everything you need to know about GridView
widget and its operation with example and output.
GridView
widget in flutter is basically a scrollable, 2D array of widgets. The most commonly used grid layouts are GridView.count
, which creates a layout with a fixed number of tiles in the cross axis, and GridView.extent
, which creates a layout with tiles that have a maximum cross-axis extent. In this example we use GridView.count
to design layout.
Code Snippet: Grid view layout in Flutter
import 'package:flutter/material.dart';
void main() => runApp(MyApp());
class MyApp extends StatelessWidget {
@override
Widget build(BuildContext context) {
return MaterialApp(
title: 'GridView Example',
home: Scaffold(
appBar: AppBar(
title: Text('GridView Example'),
centerTitle: true,
),
body: GridView.count(
primary: false,
padding: const EdgeInsets.all(4),
crossAxisSpacing: 4,
mainAxisSpacing: 4,
crossAxisCount: 3,
children: [
Container(
padding: const EdgeInsets.all(20),
child: Center(
child: const Text('FIRST'),
),
color: Colors.red,
),
Container(
padding: const EdgeInsets.all(20),
child: Center(
child: const Text('SECOND'),
),
color: Colors.indigo,
),
Container(
padding: const EdgeInsets.all(20),
child: Center(
child: const Text('THIRD'),
),
color: Colors.teal,
),
Container(
padding: const EdgeInsets.all(20),
child: Center(
child: const Text('FOURTH'),
),
color: Colors.cyan,
),
Container(
padding: const EdgeInsets.all(20),
child: Center(
child: const Text('FIFTH'),
),
color: Colors.yellow,
),
Container(
padding: const EdgeInsets.all(20),
child: Center(
child: const Text('SIXTH'),
),
),
Container(
padding: const EdgeInsets.all(20),
child: Center(
child: const Text('SEVENTH'),
),
color: Colors.purple,
),
Container(
padding: const EdgeInsets.all(20),
child: Center(
child: const Text('EIGHTH'),
),
color: Colors.blue,
),
Container(
padding: const EdgeInsets.all(20),
child: Center(
child: const Text('NINTH'),
),
color: Colors.orange,
),
],
),
),
);
}
}
Grid View Layout Output
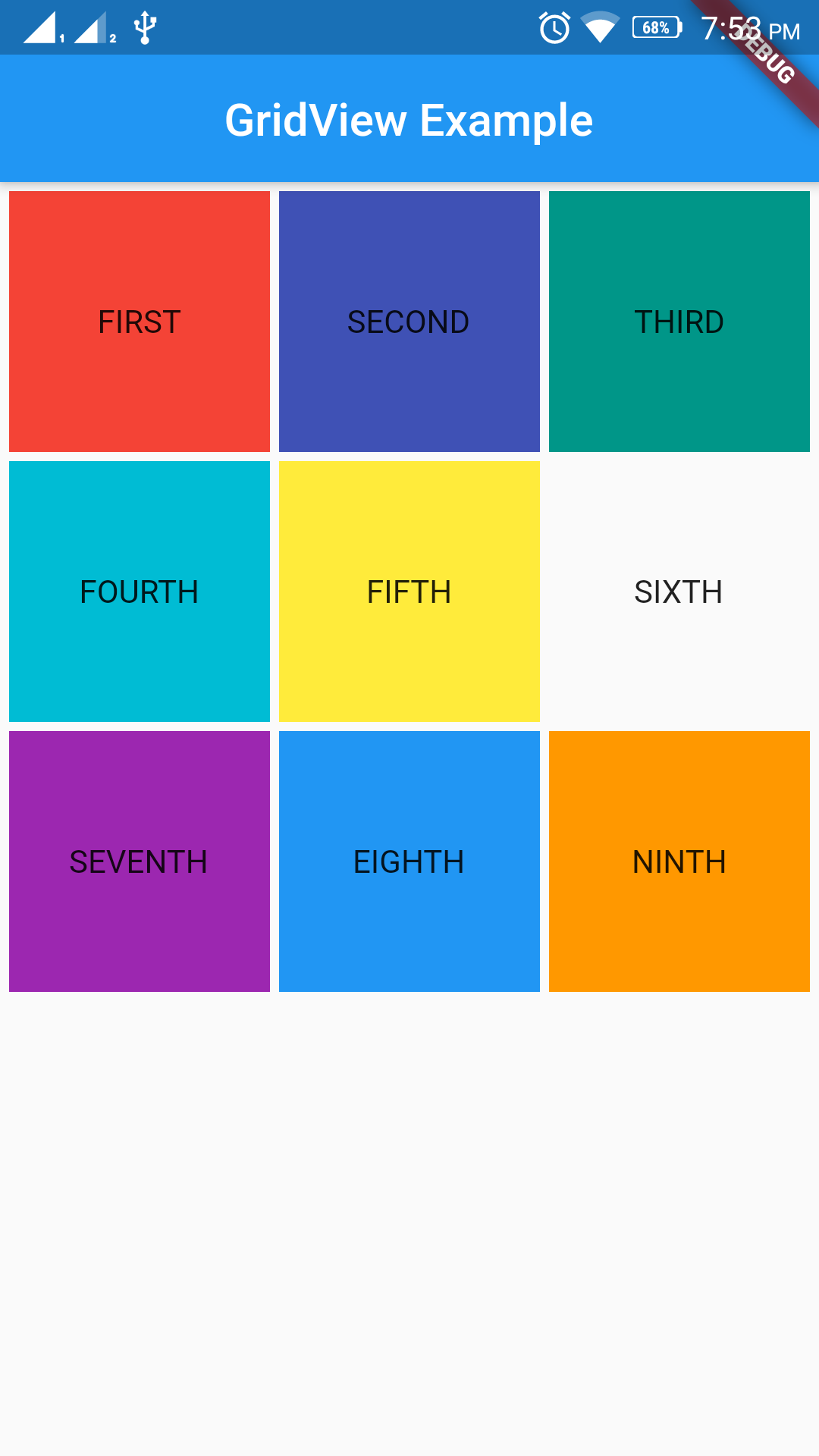
GridView Class Explanation
GridView.count
takes different arguments i.e. primary, padding, crossAxisSpacing, mainAxisSpacing, crossAxisCount, children
. Important arguments among these are:
primary:
which takes eithertrue
orfalse
and it defines whether this is the primary scroll view associated with the parent or not.mainAxisSpacing:
Spacing between elements in main axis.crossAxisSpacing:
Spacing between elements in cross axis.crossAxisCount:
Number of elements in cross axis. In this example we have three elements.children:
This is where you define different elements using widgets.
For more details please see GridView for Flutter which has documentation for this widget.