Flutter Multiple Styles in Line: Use RichText & TextSpan
In Flutter, if you need to design single line with multiple styles then it can be done by using RichText
widget with TextSpan
.
RichText
widget displays text with different styles. Different text to display is represented using a tree of TextSpan
widgts. Each of these TextSpan
widgets are associated with different style.
In this flutter example, we are going to implement simple application that displays multiple styles on same line.
Code Snippet: Text with Multiple Styles
import 'package:flutter/material.dart';
void main() => runApp(MyApp());
class MyApp extends StatelessWidget {
@override
Widget build(BuildContext context) {
return MaterialApp(
title: 'RichText Example',
home: Scaffold(
appBar: AppBar(
title: Text('RichText Example'),
centerTitle: true,
),
body: Center(
child: RichText(
text: TextSpan(
text: ' Single ',
style: TextStyle(
color: Colors.blue,
fontWeight: FontWeight.bold,
fontSize: 24,
),
children: [
TextSpan(
text: ' Line ',
style: TextStyle(
color: Colors.white,
backgroundColor: Colors.teal,
fontSize: 20,
),
),
TextSpan(
text: ' Multiple ',
style: TextStyle(
color: Colors.orange,
fontFamily: 'courier',
fontSize: 24,
),
),
TextSpan(
text: ' Styles ',
style: TextStyle(
fontStyle: FontStyle.italic,
color: Colors.pink,
fontSize: 18,
),
),
],
),
),
),
),
);
}
}
Output: Multiple Styles for Text
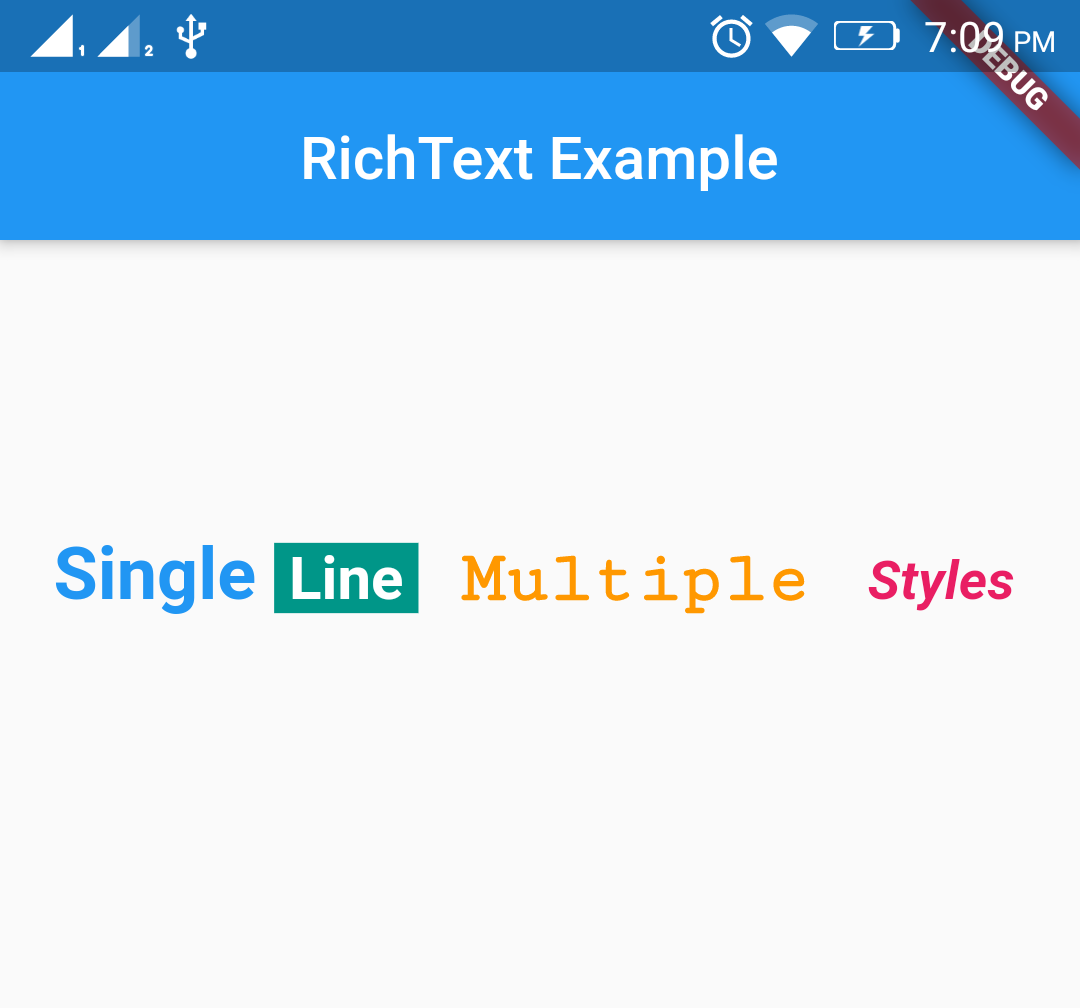
Note: Based on layout constraints the text might break across multiple lines or might all be displayed on the same line.
For more details please see RichText for Flutter which has documentation for this widget.