Pull (Swipe) to Refresh in Flutter (RefreshIndicator)
This article explains everything you need to know start with the pull-to-refresh (swipe-to-refresh) feature in your application using RefreshIndicator.
RefreshIndicator
widget supports the Material "swipe to refresh" idiom in Material Design principle.
See example below for the implementation of this feature with output.
Things to Remember: while using RefreshIndicator
widget, it's child must be Scrollable.
Code Snippet: Pull to Refresh
import 'package:flutter/material.dart';
import 'dart:async';
void main() => runApp(MyApp());
class MyApp extends StatelessWidget {
@override
Widget build(BuildContext context) {
return MaterialApp(
title: 'Pull-To-Refresh Example',
home: Scaffold(
appBar: AppBar(
title: Text('Pull-To-Refresh Example'),
centerTitle: true,
),
body: RefreshExample('At first'),
),
);
}
}
class RefreshExample extends StatefulWidget {
final String message;
RefreshExample(this.message);
@override
_RefreshExampleState createState() => _RefreshExampleState(this.message);
}
class _RefreshExampleState extends State<RefreshExample> {
String _message;
_RefreshExampleState(this._message);
@override
Widget build(BuildContext context) {
return RefreshIndicator(
child: ListView(
children: <Widget>[
ListTile(
title: Center(
child: Padding(
padding: const EdgeInsets.all(80.0),
child: Text(
_message,
style: TextStyle(
fontSize: 30.0,
),
),
),
),
),
],
),
onRefresh: () async {
Completer<Null> completer = new Completer<Null>();
await Future.delayed(Duration(seconds: 3)).then((onvalue) {
completer.complete();
setState(() {
_message = 'Refreshed';
});
});
return completer.future;
},
);
}
}
Output: Pull To Refresh
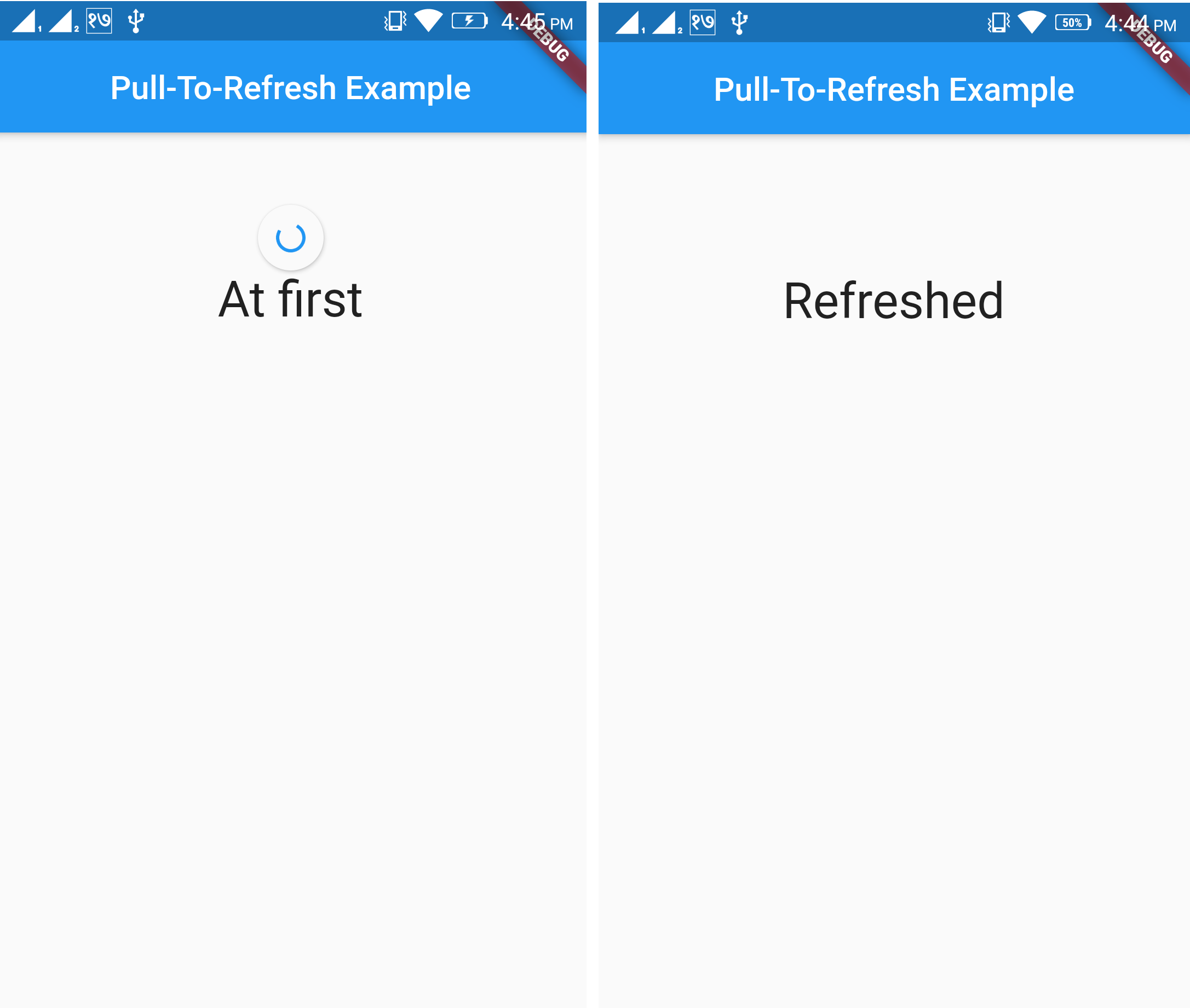
For more details please see RefreshIndicator-class which has documentation for this class.