How will you differentiate between shallow copy and deep copy in Python?
In Python there are two types of copy, shallow copy and deep copy. This article explains the difference between shallow and deep copy with examples.
Copy Using ' = ' Opeartor in Python
In Python programming, when we copy using =
operator, it does not create new objects. In this case, new variable shares the same reference of original object. So, any change made in any objects is reflected on both objects. See example below:
Example: Copy Using = Opeartor
# Copy using '=' operators
numbers = [[30, 30], [70, 70], [90, 90]]
numbers_copy = numbers
numbers_copy[0][1] = 55
print('Numbers = ', numbers)
print('Reference of numbers = ', id(numbers))
print()
print('Numbers_copy = ', numbers_copy)
print('Reference of numbers_copy = ', id(numbers_copy))
Output
Numbers = [[30, 55], [70, 70], [90, 90]] Reference of numbers = 1501470648648 Numbers_copy = [[30, 55], [70, 70], [90, 90]] Reference of numbers_copy = 1501470648648
In the above example, id
of both numbers
& numbers_copy
is same. And change made in numbers_copy
is also reflected in numbers
.
Shallow Copy
- A shallow copy creates a copy of the object but references each element of the object. In other words, shallow copy means constructing a new object and then populating it with references to the child objects found in the original object.
- The copying process is not recursive in shallow copy, so that copies of the child objects are not created.
- In case of shallow copy, a reference of object is copied into other object i.e. any changes made in copy is also reflected in the original object.
- In Python, shallow copy can be implemented by using
copy
module which hascopy()
method.
Shallow Copy Example
# shallow copy
import copy
numbers = [[30, 30], [70, 70], [90, 90]]
numbers_copy = copy.copy(numbers)
numbers_copy[0][1] = 55
print('Numbers = ', numbers)
print('Reference of numbers = ', id(numbers))
print()
print('Numbers_copy = ', numbers_copy)
print('Reference of numbers_copy = ', id(numbers_copy))
Output
Numbers = [[30, 55], [70, 70], [90, 90]] Reference of numbers = 1501470952904 Numbers_copy = [[30, 55], [70, 70], [90, 90]] Reference of numbers_copy = 1501470650120
In above example, id
of numbers
and numbers_copy
is different, but, change made in numbers_copy
is reflected in both objects.
Here is the illustration of shallow copy.
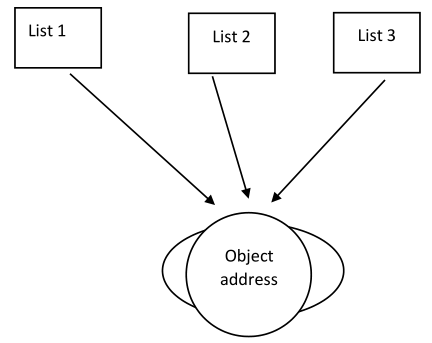
Deep Copy
- A deep copy creates a copy of the object as well as elements of the object. In other words, deep copy means first constructing a new collection object and then recursively populating it with copies of the child objects found in the original.
- The copying process is recursive in case of deep copy, hence copies of child copies are created.
- In deep copy, changes made to a copy of object do not reflect in the original object.
- In Python, shallow copy can be implemented by using
copy
module which hasdeepcopy()
method.
Deep Copy Example
# deep copy
import copy
numbers = [[30, 30], [70, 70], [90, 90]]
numbers_copy = copy.deepcopy(numbers)
numbers_copy[0][1] = 55
print('Numbers = ', numbers)
print('Reference of numbers = ', id(numbers))
print()
print('Numbers_copy = ', numbers_copy)
print('Reference of numbers_copy = ', id(numbers_copy))
Output
Numbers = [[30, 30], [70, 70], [90, 90]] Reference of numbers = 3012519166856 Numbers_copy = [[30, 55], [70, 70], [90, 90]] Reference of numbers_copy = 3012519195080
In above example, id
of numbers
and numbers_copy
is different, and, change made in numbers_copy
is not reflected in both objects.
Here is the illustration of deep copy.
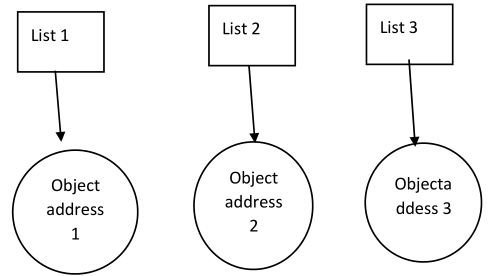
Hence, a shallow copy constructs a new compound object and then inserts references into it to the objects found in original but deep copy constucts a new compound object and then recursively inserts copies of the objects found in the original object.