do while Loop with Examples
do-while loop is post-tested loop. The do-while loop is very similar to the while loop except that the condition is checked at the end of the loop body. This guarantees that the loop is executed at least once before terminating.
do while Loop Syntax
control_variable_initialization;
do
{
statement(s);
control_variable_update;
} while (condition);
Note:control_variable_initialization
and control_variable_update
are not part of syntax of do while
loop but necessary for proper working.
do while Loop Working
Control variable is initialized first. After initialization of control variable, statements in body of loop are executed along with control variable update. After execution of statements within body of loop, condition is checked. If result of the condition evaluates to TRUE, then statements within body of loop are executed again otherwise loop will terminate.
do while Loop Flowchart
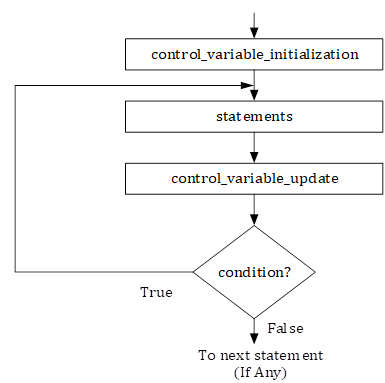
do while Loop Examples
Example 1: C program to check whether a given number is Palindrome or not using do-while.
#include<stdio.h>
#include<conio.h>
void main()
{
int num, rem, rev=0, copy;
clrscr();
printf("Enter number: ");
scanf("%d", &num);
copy = num;
do
{
rem = num%10;
rev = rev*10 + rem;
num = num/10;
} while(num!=0);
if(rev==copy)
{
printf("PALINDROME");
}
else
{
printf("NOT PALINDROME");
}
getch();
}
Output
Run1: --------------- Enter number: 1234↲ NOT PALINDROME Run2: --------------- Enter number: 1221↲ PALINDROME Run3: --------------- Enter number: 3333↲ PALINDROME Note: ↲ indicates enter is pressed.