if-else Statement with Examples
if-else statement first tests a condition then it allows us to take two possible action, one is when condition is TRUE, and the other is when condition is FALSE.
Syntax for if-else Statement
General syntax for if-else statement is:
if (Condition)
{
Statement1;
}
else
{
Statement2;
}
Note: In case of single statement within
if and else block, curly braces can
be omitted.
Working of if-else Statement
In the above general syntax if the Condition is TRUE then the Statement1 within curly braces (known as if block) is executed and control jumps to the next statements following the if-else statement. Thus, the Statement2 following the else is ignored. If the Condition is FALSE, then the Statement1 below if is ignored and the Statement2 following the else is executed. The working of if-else statement can be illustrated by following flowchart:
Flowchart for if-else Statement
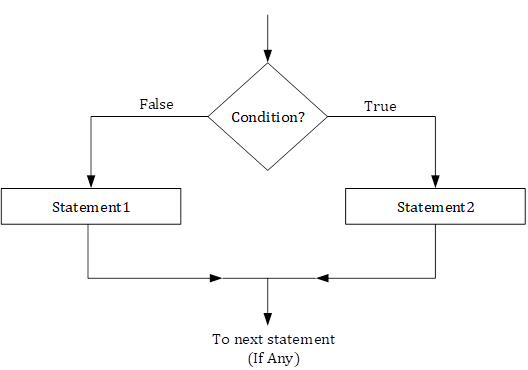
Examples for if-else Statement
Program 1: C program to check whether a given positive integer is odd or even.
#include<stdio.h>
int main()
{
int num;
printf("Enter a positive integer: ");
scanf("%d", &num);
if(num%2 == 0)
{
printf("%d is EVEN.", num);
}
else
{
printf("%d is ODD.", num);
}
return(0);
}
Output
Run 1: ------------------- Enter a positive integer: 37↲ 37 is ODD. Run 2: ------------------- Enter a positive integer: 88↲ 88 is EVEN. Note: ↲ indicates enter is pressed.
Explanation
In the first run i.e. Run 1, Value of num given by user is 37. Within if statement we have Condition num%2 == 0
, so what is happening here? We know % is modulo or remainder operator and == is relational operator for checking whether two values are equal or not. Here, num%2
is evaluated first. Since value of num is 37, 37%2 evaluates to 1. After this evaluation, condition becomes 1==0
and this gets executed. Is 1 equal to 0
? Answer is NO. So 1==0
evaluates to FALSE. When Condition in if is FALSE then statements within else are executed. In our case we have printf("%d is ODD.", num);
printing 37 is ODD.
In the second run i.e. Run 2, Value of num given by user is 88. Within if statement we have Condition num%2 == 0
, since value of num is 88, 88%2 evaluates to 0
. After this evaluation, condition becomes 0==0
and this gets executed. Is 0
equal to 0
? Answer is YES. So 0==0
evaluates to TRUE. When Condition in if is TRUE then statements within if are executed. In our case we have printf("%d is EVEN.", num);
printing 88 is EVEN.
Example 2: C program to find largest of two numbers
#include<stdio.h>
int main()
{
float a, b;
printf("Enter two numbers: \n");
scanf(" %f%f ", &a, &b);
if(a>b)
{
printf("0.1%f is Largest.", a);
}
else
{
printf("%0.1f is Largest.", b);
}
return(0);
}
Output
Run 1: ------------------- Enter two numbers: 21.5↲ 34.5↲ 34.5 is Largest. Run 2: ------------------- Enter two numbers: 78↲ -90↲ 78.0 is Largest. Note: ↲ indicates enter is pressed.
Example 3: C program to check whether a given character is ‘r’ or not.
#include<stdio.h>
int main()
{
char ch;
printf("Enter any character: ");
scanf("%c", &ch);
if(ch=='r')
{
printf("It is r.");
}
else
{
printf("It is not r.");
}
return(0);
}
Output
Run 1: ------------------- Enter any character: r↲ It is r. Run 2: ------------------- Enter any character: m↲ It is not r. Run 3: ------------------- Enter any character: +↲ It is not r. Note: ↲ indicates enter is pressed.