Ternary or Conditional Operator
Simple decision making statements in C programming can be carried out by using ternary operator (?:). The ternary operator is also known as conditional operator since it takes three operands.
The general syntax for conditional operator is:
Condition? True_Expression : False_Expresion;
Working of Ternary or Conditional Operator
In conditional operator, first Condition is evaluated to either TRUE or FALSE. If the result of Condition is TRUE then True_Expression is executed. If the result of Condition is FALSE then False_Expression is executed. Therefore, result of conditional operator is the result of whichever expression is evaluated – the True_Expression or False_Expression. Only one of the expression is evaluated in a conditional operator and never both. Following flowchart illustrates the operation of conditional operator.
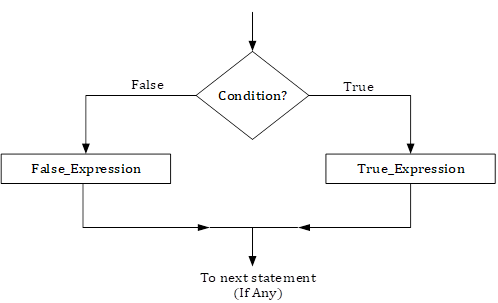
Conditional or Ternary Operator Example
Example #1 : Implementing Simple Mathematical Equation Using Conditional or Ternary Operator
#include< stdio.h >
#include< conio.h >
void main()
{
float x,y;
clrscr();
printf("Enter x: ");
scanf("%f", &x);
y = x < 0 ? x*x+4 : x*x-4;
printf("f(%f) = %f", x, y);
getch();
}
Output
Run 1: Enter x: 1.2 ↲ f(1.2) = -2.56 Run 2: Enter x: -0.5 ↲ f(-0.5) = 4.25 Note: ↲ indicates enter is pressed.
Program Explanation (Conditional or Ternary Operator)
In the above program when user enters value of x = 1.2 then condition x < 0 evaluates to FALSE. When condition evaluates to FALSE then False_Expression i.e. x*x-4 is evaluated to -2.56 and this value is assigned to y and so while printing value of y we get -2.56 in result.
Similarly, when user enters value of x = -0.5 then condition x < 0 evaluates to TRUE. When condition evaluates to TRUE then True_Expression i.e. x*x+4 is evaluated to 4.25 and this value is assigned to y and so while printing value of y we get 4.25 in result.