if-else-if Statement (Ladder) in C with Examples
In programming, if-else-if statement is also known as if-else-if ladder. It is used when there are more than two possible action based on different conditions.
Syntax
General syntax for if-else-if statement is:
if (Condition1)
{
Statement1;
}
else if(Condition2)
{
Statement2;
}
.
.
.
else if(ConditionN)
{
StatementN;
}
else
{
Default_Statement;
}
Working
In the above syntax of if-else-if, if the Condition1
is TRUE
then the Statement1
will be executed and control goes to next statement in the program following if-else-if ladder.
If Condition1
is FALSE
then Condition2
will be checked, if Condition2
is TRUE
then Statement2
will be executed and control goes to next statement in the program following if-else-if ladder.
Similarly, if Condition2
is FALSE
then next condition will be checked and the process continues.
If all the conditions in the if-else-if ladder are evaluated to FALSE
, then Default_Statement
will be executed.
The working of if-else-if ladder can be illustrated by following flowchart:
Flowchart
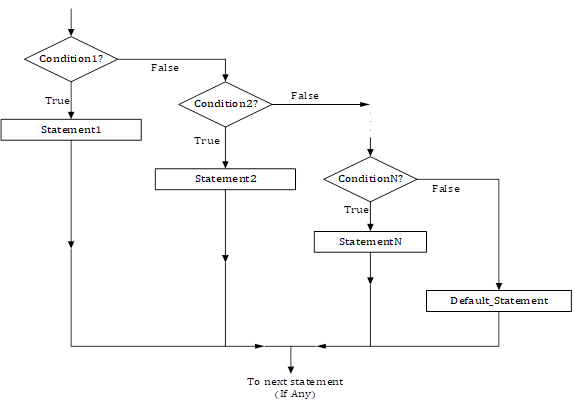
Programming Examples
Example 1: C program to find largest from three numbers given by user to explain working of if-else-if statement or ladder
#include<stdio.h>
int main()
{
int a,b,c;
printf("Enter three numbers: \n");
scanf("%d%d%d", &a, &b, &c);
if(a>b && a>c)
{
printf("Largest = %d", a);
}
else if(b>a && b>c)
{
printf("Largest = %d", b);
}
else
{
printf("Largest = %d", c);
}
return(0);
}
Output
Run 1: ------------- Enter three numbers: 12 ↲ 33 ↲ -17 ↲ Largest = 33 Run 2: ------------- Enter three numbers: -18 ↲ -31 ↲ -17 ↲ Largest = -17 Run 3: ------------- Enter three numbers: 118 ↲ 19 ↲ -87 ↲ Largest = 118 Note: ↲ indicates enter is pressed.
Explanation
During the first run i.e. Run 1, numbers given by user are 12, 33, and -17 respectively. These numbers are stored in variable a, b and c i.e. a gets 12, b gets 33 and c gets -17. In the if-else-if ladder first condition is a>b && a>c
. So, what happens here? Lets break this condition!
Putting values of a, b and c condition becomes 12>33 && 12>-17
, Right? In the first part of condition, we have 12>33. This is checking, is 12 greater than 33? Answer is NO. So, 12>33
evaluates to FALSE. Similarly, In the second part of condition, we have 12>-17. This is checking, is 12 greater than -17? Answer is YES. So, 12>-17
evaluates to TRUE. Results of these two conditions are combined by logical AND opeartor. So, finally FALSE && TRUE
is evaluated. Since logical AND opeartors gives FALSE when one of the operand is FALSE, so FALSE && TRUE
evaluates to FALSE.
When condition in if is FALSE then it prevents execution of printf("Largest = %d", a);
and checks for another condition. Another condition here is b>a && b>c
. Which is TRUE && TRUE
, which in turn evaluates to TRUE
. So b>a && b>c
evaluates to TRUE and hence printf("Largest = %d", b);
is executed printing Largest = 33. And then execution goes to next statement neglecting else block where it executes retun(0)
which is the last statement in the program and program execution comes to end.
Example 2: C program to print weekday based on given number.
#include<stdio.h>
int main()
{
int day;
printf("Enter day number: ");
scanf("%d", &day);
if(day==1)
{
printf("SUNDAY.");
}
else if(day==2)
{
printf("MONDAY.");
}
else if(day==3)
{
printf("TUESDAY.");
}
else if(day==4)
{
printf("WEDNESDAY.");
}
else if(day==5)
{
printf("THURSDAY.");
}
else if(day==6)
{
printf("FRIDAY.");
}
else if(day==7)
{
printf("SATURDAY.");
}
else
{
printf("INVALID DAY.");
}
return(0);
}
Output:
Run 1: ------------- Enter day number: 4↲ WEDNESDAY. Run 2: ------------- Enter day number: 2↲ MONDAY. Run 3: ------------- Enter day number: 10↲ INVALID DAY. Run 4: ------------- Enter day number: -1↲ INVALID DAY. Note: ↲ indicates enter is pressed.
Example 3: C program to find largest number from four given numbers.
#include<stdio.h>
int main()
{
float a,b,c,d, lg;
printf("Enter four numbers:\n");
scanf("%f%f%f%f", &a, &b, &c, &d);
if(a>b && a>c && a>d)
{
lg = a;
}
else if(b>a && b>c && b>d)
{
lg = b;
}
else if(c>a && c>b && c>d)
{
lg = c;
}
else
{
lg = d;
}
printf("Largest = %f", lg);
return(0);
}
Output
Run 1: ------------- Enter four numbers: 21.4↲ 54.4↲ 34.5↲ -67.5↲ Largest = 54.400000 Note: ↲ indicates enter is pressed.