for Loop with Examples
The for loop is most commonly used looping statement. It is pre-tested loop and it is used when the number of repetitions (iterations) are known in advance. The syntax of the loop consists three parts, they are, control variable initialization, condition and control variable update. These three parts are separated by semicolon.
for Loop Syntax
for (control_variable_initialization;condition;control_variable_update)
{
statement(s); //body of loop
}
Note: Curly braces are optional if body of
loop consists single statement.
for Loop Working
In for loop control variable is initialized first. After initialization of control variable, it tests condition. Based on the outcome of condition, loop is continued or stopped. If the condition evaluates to FALSE, then loop will be terminated (goes to next statement following loop). If the condition evaluates to TRUE, then statements within body of loop are executed. After execution of statements, value of loop control variable is updated and condition is checked again. And the process is repeated. Following flowchart illustrates the operation of for loop.
for Loop Flowchart
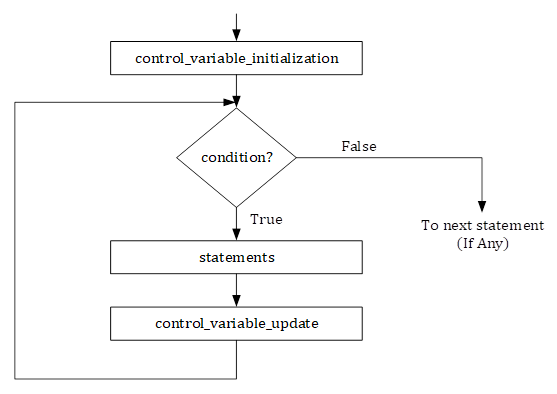
for Loop Examples
Example 1: Program to print “Programming is fun.” 10 times to explain working of for loop
#include<stdio.h>
int main()
{
int i;
for(i=1;i<=10; i++)
{
printf("Programming is fun.\n");
}
return(0);
}
Output of Above Example to Explain Working of for Loop
Programming is fun. Programming is fun. Programming is fun. Programming is fun. Programming is fun. Programming is fun. Programming is fun. Programming is fun. Programming is fun. Programming is fun.
Example 2: Program to print “Programming is fun.” n times, where n is given by user
#include<stdio.h>
int main()
{
int i, n;
printf("Enter n: ");
scanf("%d", &n);
for(i=1;i<=n; i++)
{
printf("Programming is fun.\n");
}
return(0);
}
Output
Enter n: 5 ↲ Programming is fun. Programming is fun. Programming is fun. Programming is fun. Programming is fun. Note: ↲ indicates enter is pressed.
Example 3: Program to find sum and average of n numbers given by user
#include<stdio.h>
int main()
{
int i, n;
float sum=0, avg, num;
printf("Enter n: ");
scanf("%d", &n);
for(i=1;i<=n; i++)
{
printf("Enter number-%d:",i);
scanf("%f",&num);
sum = sum + num;
}
avg = sum/n;
printf("Sum is %f\n", sum);
printf("Average is %f", avg);
return(0);
}
Output
Enter n: 4 ↲ Enter number-1: 12 ↲ Enter number-2: 21 ↲ Enter number-3: 14 ↲ Enter number-4: 47 ↲ Sum is 94 Average is 23.5 Note: ↲ indicates enter is pressed.