Recursive Function in C Programming (Recursion)
In C programming, a function is allowed to call itself. A function which calls itself directly or indirectly again and again until some specified condition is satisfied is known as Recursive Function.
A recursive function is a function defined in terms of itself via self-calling expressions. This means that the function will continue to call itself and repeat its behavior until some condition is satisfied to return a value.
Recursion is the process of defining something in terms of itself. Recursion has many negatives. It repeatedly invokes the mechanism, and consequently increases the overhead of function calls. This repetition can be expensive in terms of both processor time and memory space.
Each recursive call causes another copies of the function, these set of copies can consume considerable memory space.
Recursive Function Example
Program to find factorial of a given number recursively to illustrate recursive function
/*
Program: Factorial of a given number using recursive function.
Author: Codesansar
Date: Jan 22, 2018
*/
#include<stdio.h>
#include<conio.h>
int fact(int n); /* Function Definition */
void main()
{
int num, res;
clrscr();
printf("Enter positive integer: ");
scanf("%d",&num);
res = fact(num); /* Normal Function Call */
printf("%d! = %d" ,num ,res);
getch();
}
int fact(int n) /* Function Definition */
{
int f=1;
if(n <= 0)
{
return(1);
}
else
{
f = n * fact(n-1); /* Recursive Function Call as fact() calls itself */
return(f);
}
}
Output
Enter positive integer: 5↲ 5! = 120
Working of above recursive example can be illustrated using following diagrams:
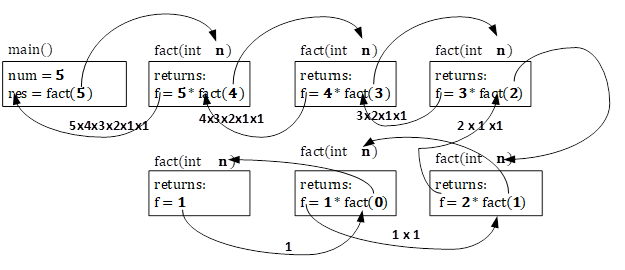