switch Statement with Examples
switch Statement first evaluates expression that is used with switch statement. Then, the control is transferred to one of the several alternatives that matches the case constant. The value of expression can be of type int and char
but not of type float or double
.
Syntax for switch Statement
General syntax for switch statement is:
switch (Expression)
{
case Constant1: Statement1;
break;
case Constant2: Statement2;
break;
.
.
.
case ConstantN: StatementN;
break;
default: Default Statement;
break;
}
Rules that must be remembered while using switch statement:
- Expression and constant must be of type either integer or character.
- All the cases must be distinct.
- The block of statement under default will be executed when none of the cases match the value of expression.
- The
break
statement causes exit from switch statement. If it is not present, after executing the case which match the value of the expression, the following other cases are executed.
switch Statement Working
It first evaluates expression that is used with switch statement. After evaluating expression, expression value is matched with different case constants. Then the control is transferred to one of the several alternatives that matches the case constant. For example, if value of evaluated expression is equal to Constant1 then Statement1 will be executed. Similarly, if it matches to Constant2, then Statement2 is executed. The value of expression can be of type int and char
but not of type float or double
. When break
is encountered within switch then control comes out from switch statement.
switch Statement Flowchart
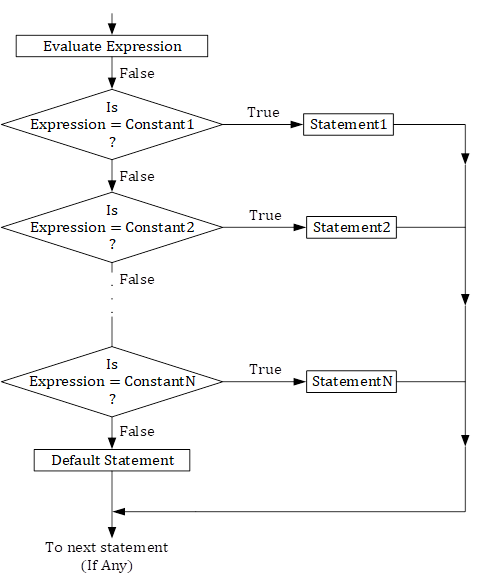
Examples for switch Statement
Example 1: C program to check whether a given number is odd or even using switch statement
#include<stdio.h>
int main()
{
int num, rem;
printf("Enter positive integer: ");
scanf("%d", &num);
rem = num%2;
switch(rem)
{
case 1: printf("%d is ODD.", num);
break;
default: printf("%d is EVEN.", num);
break;
}
return(0);
}
Output of Above switch statement example
Run 1: ------------------ Enter positive integer: 21↲ 21 is ODD. Run 2: ------------------ Enter positive integer: 66↲ 66 is EVEN. Note: ↲ indicates enter is pressed.
Explanation
In the above example, in the first run number given by user is stored in variable num (here given number is 21) and then remainder is calculated dividing given number by 2. After this operation rem = 21 % 2 = 1
. And in the switch statement switch(1)
is executed. Now switch transfers the control to one of the matching case. Here it matches with case 1
. And in the case 1 statement printf("%d is ODD", num);
is executed giving output 21 is ODD.
Similarly, in the second run number given by user is stored in variable num (here given number is 66) and then remainder is calculated dividing given number by 2. After this operation rem = 66 % 2 = 0
. And in the switch statement switch(0)
is executed. Now switch transfers the control to one of the matching case. BUT!, here it does not have any matching case. When there is no matching case then control goes to default
. And in the default section statement printf("%d is EVEN", num);
is executed giving output 66 is EVEN.
Example 2: C program to print weekday based on given number using switch
#include<stdio.h>
int main()
{
int day;
printf("Enter day number: ");
scanf("%d", &day);
switch(day)
{
case 1: printf("SUNDAY.");
break;
case 2: printf("MONDAY.");
break;
case 3: printf("TUESDAY.");
break;
case 4: printf("WEDNESDAY.");
break;
case 5: printf("THURSDAY.");
break;
case 6: printf("FRIDAY.");
break;
case 7: printf("SATURDAY.");
break;
default: printf("INVALID DAY.");
break;
}
return(0);
}
Output
Run 1: ------------------ Enter day number: 3↲ TUESDAY. Run 2: ------------------ Enter day number: -3↲ INVALID DAY. Run 3: ------------------ Enter day number: 6↲ FRIDAY. Note: ↲ indicates enter is pressed.
Example 3: C program to implement simple calculator using switch statement
#include<stdio.h>
int main()
{
float a,b, r;
char op;
printf("Available Operations.\n");
printf("+ for Addition.\n");
printf("- for Subtraction.\n");
printf("* for Multiplication.\n");
printf("/ for Division.\n");
printf("Which Operation?\n");
scanf("%c", &op);
switch(op)
{
case '+': printf("Enter two numbers:\n");
scanf("%f%f",&a, &b);
r = a+b;
printf("%f + %f = %f", a, b, r);
break;
case '-': printf("Enter two numbers:\n");
scanf("%f%f",&a, &b);
r = a-b;
printf("%f - %f = %f", a, b, r);
break;
case '*': printf("Enter two numbers:\n");
scanf("%f%f",&a, &b);
r = a*b;
printf("%f * %f = %f", a, b, r);
break;
case '/': printf("Enter two numbers:\n");
scanf("%f%f",&a, &b);
if(b!=0)
{
r = a/b;
printf("%f/%f = %f",a,b,r);
}
else
{
printf("Division not possible.");
}
break;
default: printf("Invalid Operation.");
break;
}
return(0);
}
Output
Run 1: ------------------ Available Operations. + for Addition. - for Subtraction. * for Multiplication. / for Division. Which Operation? * ↲ Enter two numbers: 13↲ 17↲ 13 * 17 = 221 Run 2: ------------------ Available Operations. + for Addition. - for Subtraction. * for Multiplication. / for Division. Which Operation? ^↲ Invalid Operation. Run 3: ------------------ Available Operations. + for Addition. - for Subtraction. * for Multiplication. / for Division. Which Operation? /↲ Enter two numbers: 13↲ 17↲ 12/21 = 0.571429 Run 4: ------------------ Available Operations. + for Addition. - for Subtraction. * for Multiplication. / for Division. Which Operation? /↲ Enter two numbers: 13↲ 0↲ Division not possible. Note: ↲ indicates enter is pressed.