Nested if-else Statement with Examples
In C programming, we can have if-else statement within another if-else statement. When if-else statement comes within another if-else statement then this is known nesting of if-else statement.
Syntax for Nested if-else Statement
if (Condition1)
{
if(Condition2)
{
Statement1;
}
else
{
Statement2;
}
}
else
{
if(Condition3)
{
Statement3;
}
else
{
Statement4;
}
}
Working of Nested if-else Statement
In this example of nested if-else, it first tests Condition1, if this condition is TRUE then it tests Condition2, if Condition2 evaluates to TRUE then Statement1 is executed otherwise Statement2 will be executed. Similarly, if Condition1 is FALSE then it tests Condition3, if Condition3 evaluates to TRUE then Statement3 is executed otherwise Statement4 is executed. The working of nested if-else presented in this example can be further illustrated by following flowchart.
Flowchart for Nested if-else Statement
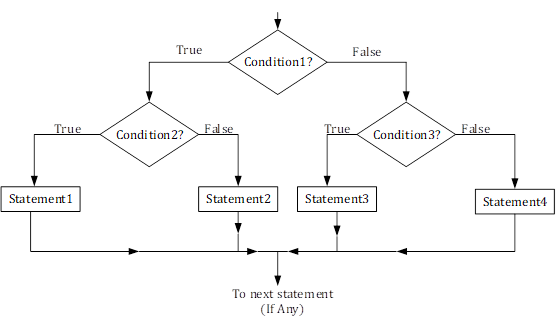
Examples for Nested if-else Statement
Example 1: C program to find largest from three numbers given by user to Explain Nested if-else
#include<stdio.h>
int main()
{
int num1, num2, num3;
printf("Enter three numbers:\n");
scanf("%d%d%d",&num1, &num2, &num3);
if(num1>num2)
{
/* This is nested if-else */
if(num1>num3)
{
printf("Largest = %d", num1);
}
else
{
printf("Largest = %d", num3);
}
}
else
{
/* This is nested if-else */
if(num2>num3)
{
printf("Largest = %d", num2);
}
else
{
printf("Largest = %d", num3);
}
}
return(0);
}
Output of Above Program to Illustrate Nested if-else
Run 1: --------------- Enter three numbers: 12↲ 33↲ -17↲ Largest = 33 Run 2: --------------- Enter three numbers: -18↲ -31↲ -17↲ Largest = -17 Run 3: --------------- Enter three numbers: 118↲ 19↲ -87↲ Largest = 118 Note: ↲ indicates enter is pressed.
Explanation
In the above program to illustrate concept of nested if-else statement, first values of num1, num2 and num3 are read from users and these values are 12, 33 and -17 respectively. After reading these values first condition num1 > num2
is checked. Since 12 > 33
is FALSE so control goes to outer else block. When control goes to outer else block then num2 is greater. Within outer else block condition num2 > num3
is checked. Since this if-else block is within another if-else block so this is nested if-else. Here condition 33 > -17
is TRUE so control goes to if block within outer else block i.e. printf("Largest = %d", num3);
is executed giving output Largest = 33.