if Statement with Examples
if statement is very useful and easy to use for decision making. It allows a particular statement or more than one statements (block of statements) to be executed only when certain condition is fulfilled.
if Statement Syantax
General syntax for if statement is :
if (Condition)
{
Statements;
}
Note: In case of single statement within
if block, curly braces can be omitted.
if Statement Working
In the above general syntax if the Condition is TRUE then the Statements within curly braces (known as if block) is executed and control jumps to the next statements following the if statement. If Condition is FALSE then statements within if block are not executed and control goes to next statements. The working of if statement can be illustrated by following flowchart:
if Statement Flowchart
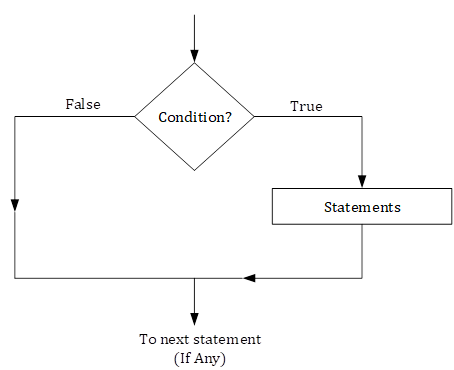
if Statement Examples
Example #1 : C Program to Explain Working of if Statement
#include<stdio.h>
int main()
{
int a;
printf("Enter a number: ");
scanf("%d", &a);
if(a > 10)
{
printf("In if statement.\n");
}
printf("Welcome to C programming.");
return(0);
}
Output of above program :
Run 1: ------------------- Enter a number: 50 ↲ In if statement. Welcome to C programming. Run 2: ------------------- Enter a number: 5 ↲ Welcome to C programming. Note: ↲ indicates enter is pressed.
Explanation of above example :
In the above example of if statement, in the first run i.e. Run 1, value of a given by user is 50. This value is checked for whether it is greater than 10 or not in Condition of if statement. Since 50 is greater than 10 so Condition a>50 evaluates to TRUE and statements printf("In if statement\n.");
is executed. Since there are no other statements within if block so control comes out from if block. When control comes out from if block then it finds statement printf("Welcome to C Programming");
is executed. And program execution comes to end since there are no others statement left to execute.
In the above example of if statement, in the second run i.e. Run 2, value of a given by user is 5. This value is checked for whether it is greater than 10 or not in Condition of if statement. Since 5 is not greater than 10 so Condition a>5 evaluates to FALSE and statements printf("In if statement\n.");
is not executed i.e. execution does not enter to if block. Now control goes to next statement following if and next statement here is printf("Welcome to C Programming");
. This gets executed. And program execution comes to end since there are no others statement left to execute.
Let us consider another example to read a number from user and to increase it by 15 if it is greater than 8 otherwise to keep as it is.
Example #2: C Program to increase number by 15 if it is greater than 8 otherwise to keep as it is
#include<stdio.h>
int main()
{
int num;
printf("Enter a number: ");
scanf("%d", &num);
if(num>8)
{
num = num + 15;
}
printf("Output is %d", num);
return(0);
}
Output of above program :
Run 1: ------------------- Enter a number: 10 ↲ Output is 25 Run 2: ------------------- Enter a number: -5 ↲ Output is -5 Note: ↲ indicates enter is pressed.