while Loop with Examples
while loop is pre-tested loop, it tests the expression before every repetition of the loop body. Statements within body of loop are executed until some condition is satisfied. The while loop looks like simplified version of the for loop. In its syntax it consists condition but not control variable initialization and control variable update. However, loop control variable must be initialized before condition is checked and loop body must also contain control variable update to avoid infinite loop.
while Loop Syntax
General syntax for while loop is:
control_variable_initialization;
while (condition)
{
statement(s);
control_variable_update;
}
---------------------------------------------
Note 1: control_variable_initialization and
control_variable_update are not part of syntax
of while loop but necessary for proper working.
Note 2:Curly braces are optional if loop body
consist single statement.
while Loop Working
In while loop control variable is initialized first. After initialization of loop control variable, it tests condition. Based on the result of test condition, loop is continued or stopped. If condition evaluates to FALSE, then loop will be terminated (goes to next statement following loop). If condition evaluates to TRUE, then statements within body of loop are executed along with control variable update. After execution of body of loop, condition is checked again. And the process is repeated. Following flowchart illustrates the operation of while loop.
while Loop Flowchart
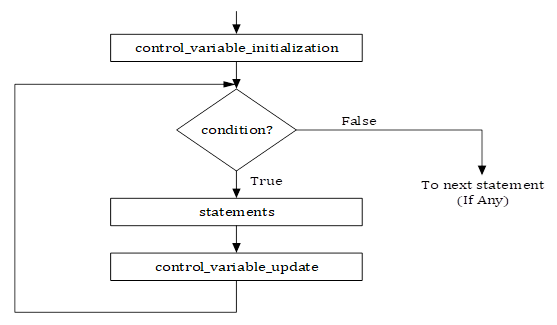
while Loop Examples
Example #1: Program to display 1 to 10 using while loop.
#include<stdio.h>
#include<conio.h>
void main()
{
int i;
clrscr();
i=1;
while(i<=10)
{
printf("%d\n",i);
i++;
}
getch();
}
Output
1 2 3 4 5 6 7 8 9 10
Example #2: Program to find sum of digit of a given number.
#include<stdio.h>
#include<conio.h>
void main()
{
int num, rem, sum=0;
clrscr();
printf("Enter number: ");
scanf("%d", &num);
while(num!=0)
{
rem = num%10;
sum = sum + rem;
num = num/10;
}
printf("Sum is %d", sum);
getch();
}
Output
Run1: --------------- Enter number: 15234↲ Sum is 15 Run2: --------------- Enter number: 6878↲ Sum is 29 Note: ↲ indicates enter is pressed.
Example #3: Program to reverse a given number.
#include<stdio.h>
#include<conio.h>
void main()
{
int num, rem, rev=0;
clrscr();
printf("Enter number: ");
scanf("%d", &num);
while(num!=0)
{
rem = num%10;
rev = rev*10 + rem;
num = num/10;
}
printf("Reverse is %d", rev);
getch();
}
Output
Run1: --------------- Enter number: 1234↲ Reverse is 4321 Run2: --------------- Enter number: 6878↲ Reverse is 8786 Note: ↲ indicates enter is pressed.