Python Program to Plot a Circle Using Numpy & Matplotlib
This Python programming example plots a circle using Numpy and Matplotlib Library.
In this program, we use numpy
to generate data for theta, x and y co-ordinates and pyplot
from matplotlib
is used to plot data.
This example uses formula x = r*cos(θ) and y = r * sin(θ) to generate x and y co-ordinates.
Python Source Code: Circle Plot
# Python program to Plot Circle
# importing libraries
import numpy as np
from matplotlib import pyplot as plt
# Creating equally spaced 100 data in range 0 to 2*pi
theta = np.linspace(0, 2 * np.pi, 100)
# Setting radius
radius = 5
# Generating x and y data
x = radius * np.cos(theta)
y = radius * np.sin(theta)
# Plotting
plt.plot(x, y)
plt.axis('equal')
plt.title('Circle')
plt.show()
Output
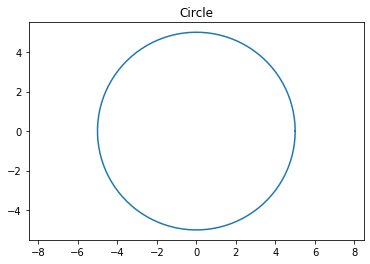