Python Program to Plot Limacon Using Numpy & Matplotlib
This Python program plots Limacon using Numpy and Matplotlib. To plot limacon we use polar()
method from matplotlib.pytplot
.
In mathematics, Limacon has general form of r = a ± b cos(θ) or r = a ± b sin(θ).
In this Python example, we are going to plot polar curve for Cardioid, Dimpled Limacon, Limacon with no dimple & no inner loop and Limacon with inner loop.
Dimpled Limacon - Python Polar Plot
Dimpled Limacon has general form r = a ± bcos(θ) or r = a ± b sin(θ) with 1 < a/b < 2.
For example, if we plot r = 3 + 2 * cos(θ), it gives Dimpled Limacon since it has a = 3 and b = 2 which gives a/b = 3/2 = 1.5 is between 1 and 2.
Python Source Code: Dimpled Limacon
# Python program to plot Dimpled Limacon
import numpy as np
from matplotlib import pyplot as plt
theta = np.linspace(0, 2*np.pi, 1000)
r = 3 + 2 * np.cos(theta)
plt.polar(theta, r)
plt.show()
Output
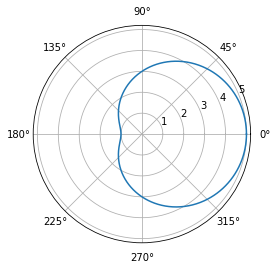
Cardioid Limacon - Python Polar Plot
Cardioid Limacon has general form r = a ± bcos(θ) or r = a ± b sin(θ) with a/b = 1.
For example, r = 4 + 4 * np.sin(θ) produces Cardioid Limacon.
Python Source Code: Cardioid Limacon
# Python program to plot Cardioid Limacon
import numpy as np
from matplotlib import pyplot as plt
theta = np.linspace(0, 2*np.pi, 1000)
r = 4 + 4 * np.sin(theta)
plt.polar(theta, r)
plt.show()
Output
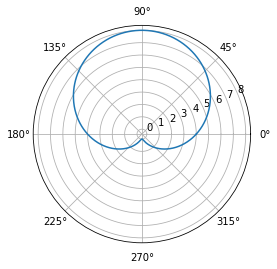
Limacon With No Dimple & No Inner Loop
Limacon with no dimple and no inner loop has general form r = a ± bcos(θ) or r = a ± b sin(θ) with a/b ≥ 2.
For example, r = 7 - 2 * np.cos(θ) produces Limacon with no dimple and no inner loop.
Python Source Code
# Python program to plot Limacon with ni inner loop and no dimple
import numpy as np
from matplotlib import pyplot as plt
theta = np.linspace(0, 2*np.pi, 1000)
r = 7 - 2 * np.cos(theta)
plt.polar(theta, r)
plt.show()
Output
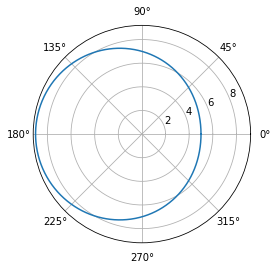
Limacon With Inner Loop - Python Polar Plot
Limacon with inner loop has general form r = a ± bcos(θ) or r = a ± b sin(θ) with a/b < 1.
Likewise, if we plot any equation satisfying above condition we will get Limacon with inner loop.