Python Program to Plot Growing & Decaying Exponential Curve
This Python program plots growing and decaying exponential curve using numpy and matplotlib library.
Python Source Code: Exponential Function
# Importing Required Libraries
import numpy as np
import matplotlib.pyplot as plt
# Generating time data using arange function from numpy
time = np.arange(-2,2, 0.0001)
constant = 0.8
# Finding amplitude at each time
amplitude_grow = constant * np.exp(time)
amplitude_decay = constant * np.exp(-time)
# Plotting time vs amplitude using plot function from pyplot
plt.plot(time, amplitude_grow, time, amplitude_decay)
# Settng title for the plot in blue color
plt.title('Exponential Curve', color='b')
# Setting x axis label for the plot
plt.xlabel('Time'+ r'$\rightarrow$')
# Setting y axis label for the plot
plt.ylabel('Amplitude '+ r'$\rightarrow$')
# Showing legends
plt.legend(['Growing Exponential','Decaying Exponential'])
# Showing grid
plt.grid()
# Highlighting axis at x=0 and y=0
plt.axhline(y=0, color='k')
plt.axvline(x=0, color='k')
# Finally displaying the plot
plt.show()
Output
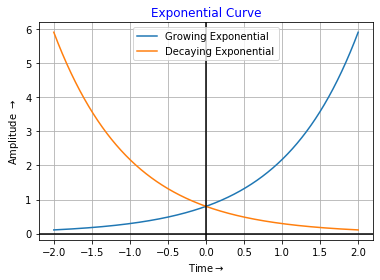